In this tutorial, we will learn how to get data from two models in Laravel. We are explaining with very easy examples which you can easily understand. You can easily apply it with your laravel 5, laravel 6, laravel 7, and laravel 8 application.
We have two options to Get data from different models in laravel, The first one is to get data and use 2 foreach loops in the Blade file, and the second one is to Merge these models in the controller and get data using only a single variable. So here we will try to achieve this by merging two models in the controller and get the data in one.
In the MVC framework, the letter “M” stands for Model. Model are means to handle the business logic in any MVC framework-based application. In Laravel, Model is a class that represents the logical structure and relationship of the underlying data table. In Laravel, each database table has a corresponding “Model” that allows us to interact with that table. Models give you the way to retrieve, insert, and update information into your data table. All of the Laravel Models are stored in the main app directory.
Sometimes we need to get the data from two tables using the model. We use model because it is a very convenient and easy to get data from the database. So let’s suppose we need to get the data from two tables from two different models, how can we achieve that. Well, that is possible and we have explained that in the below example.
Example
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Parentuser; use App\Models\Student; class TestController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $parent = Parentuser::get(); $student = Student::get(); $data = $parent->concat($student); dd($data); } }
You can call two models using namespace in the controller and concat them as defined in the above example.
Output:
Illuminate\Database\Eloquent\Collection {#281 ?
#items: array:4 [?
0 => App\Models\Parentuser {#297 ?}
1 => App\Models\Parentuser {#298 ?}
2 => App\Models\Student {#1015 ?}
3 => App\Models\Student {#1014 ?}
]
}
Table 1:
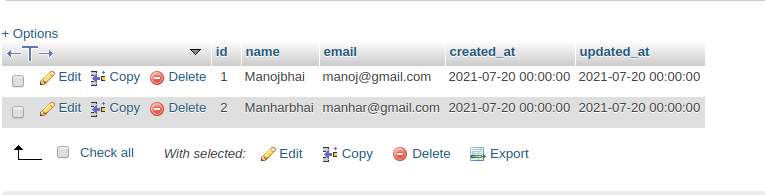
Table 2:
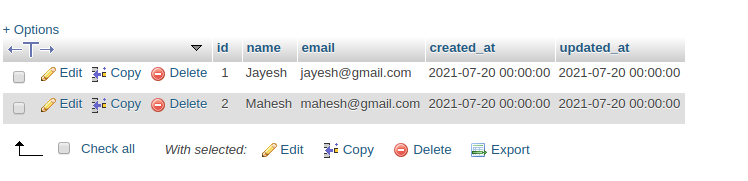
Hope this tutorial has solved your query in the easy possible way.