In this post, we will learn how to Remove multiple elements from an array using Javascript or jQuery give with an example. We can simply remove elements from an array using javascript. Below in the post, we have tried different methods to do the same.
Let’s suppose we have an array in which and we want to remove some particular element from the array without refreshing the page. In that case, we have to use javascript and jquery to remove the element from the array. Because if we go for server-side language then we have to refresh the page or need to get done the same thing with the help of AJAX and backend language. Which is a kind of long and boring task to do. We can simply use javascript, jquery, and some of the other javascript libraries to do it.
Following are the some example we are providing to make you understand about the topic.
Remove element using Vanilla JavaScript
In first, let’s remove the element using simple javascript and jquery, In this, we will use Array.prototype.remove, grep() and inArray() to remove multiple as well as the duplicate value from the array in jquery. However, you can check How to remove duplicate values from an array in PHP
<!DOCTYPE html> <html> <head> <title>Jquery Remove Multiple Values from Array Example</title> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> </head> <body> <script type="text/javascript"> var sites = [ "website1.com", "website2.com", "website3.com", "website4.com" ]; Array.prototype.remove = function(){ var args = Array.apply(null, arguments); return $.grep(this, function(value) { return $.inArray(value, args) < 0; }); } sitesNew = sites.remove("website2.com", "website3.com"); console.log(sitesNew); </script> </body> </html>
Output
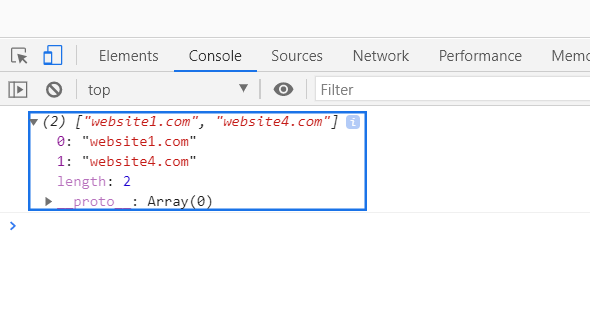
Remove element using Using Lodash
To remove an element, we can use an external javascript library called Lodash. Lodash has the remove()
method which is very easy to use. All other methods in the Lodash are very easy to use and helpful which can done job for you in a second. You do not need to break the array and manipulate for getting some work done in javascript. It have very helpful built in methods which you can use in any situation in the programming.
In the below code we are just passing the array in _.remove() and in which we have defined callback function when passed argument is == to 3.
NOTE : It make the changes in the existing array value.
var arr = [1, 2, 3, 3, 4, 5]; _.remove(arr, function(e) { return e === 3; }); console.log(arr); // Output: // [ 1, 2, 4, 5 ]
Remove element using Using Underscore
We can use Underscore library to solve the same problem. It has _.reject method which is same like _.remove() method in the Lodash. It works similarly like Lodash with some difference which is : it returns the new array after removing the passed value in the array. Old array value remains same.
See the following code for an example:
var arr = [1, 2, 3, 3, 4, 5]; var ret = _.reject(arr, function(e) { return e === 3; }); console.log(arr); console.log(ret); // Output: // [ 1, 2, 3, 3, 4, 5 ] // [ 1, 2, 4, 5 ]
Conclusion
We have explained 3 different method and by using them you can remove the one or more elements from the array in simple way. I hope you guys have liked the post, if you have any suggestion please comment below., i would love to help you.