What Is a Route?
Route is a way of creating a URL in Laravel. You can make as much as possible URL in Laravel and for that, you do not need to map URLs to specific files on a website. These URL is are both human-readable and SEO friendly
In Laravel, routes are managed and created in the routes folder. If we need to make the URL for the website we create it in web.php and similarly we create Routes for the API in api.php.
We can divide routing in the following categories –
- Basic Routing
- Route parameters
- Named Routes
Basic Routing
Routes are registered in the routes/web.php file. when the user hits the URL, This file tells Laravel to respond to the associated controller to this URL and controller work as per defined function in it.
The following is the sample route for the welcome page and screenshot to locate the route file in the Laravel application.
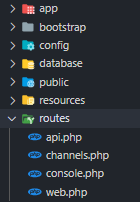
Route code in Web.php file.
Route::get ('/', function () { return view('welcome'); });
So basically Route is a class somewhere in Laravel and get is a method defined in that class. Like, get we have multiple methods in Laravel.
Available Router Methods
Route::get($uri, $callback); Route::post($uri, $callback); Route::put($uri, $callback); Route::patch($uri, $callback); Route::delete($uri, $callback); Route::options($uri, $callback);
Example
Observe the following example to understand more about Routing −
routes/web.php
<?php Route::get('/', function () { return view('welcome'); });
resources/view/welcome.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel</title> <link href = "https://fonts.googleapis.com/css?family=Lato:100" rel = "stylesheet" type = "text/css"> <style> html, body { height: 100%; } body { margin: 0; padding: 0; width: 100%; display: table; font-weight: 100; font-family: 'Lato'; } </head> <body> <div class = "container"> <div class = "content"> <div class = "title">Welcome</div> </div> </div> </body> </html>
When the URL is called, it should match the method in web.php. In the above example, the request should match (‘/’) URL. Once the request matched it will return the welcome view in resources/views/welcome.blade.php.
Route Parameters
Sometimes we need to pass the extra variable in the URL when required. You can pass the parameters in web.php file in two ways.
Required Parameters
The following is an example where you can see we have passed the id parameter in the URL. So whenever the user hits that name URL it should contain the id in that, otherwise, it will throw an error.
Route::get('name/{id}',function($id) { echo 'name: '.$id; });
Optional Parameters
We have seen in the above paragraph to pass the value in the URL and which is mandatory. But you can make it optional with the inclusion of ? after the parameter name as employeeName?
We have to provide it a default value, When we don’t get any parameters in the URL, the function will return the default value, You can mention NULL also.
Route::get('EmployeeData/{employeeName?}', function ($employeeName= 'defaultValue'){ return $employeeName; });
Named Routes
Named routes allow us to generate convenient routes names. We can chain the route and using the name method we can define the name for that particular route. Which you can use further in the controller to redirect and call.
Route::get('employee/profile', 'EmployeeController@displayProfile')->name('employeeProfile');