In this article, we are going to discuss, how we can send push notification from the website using Firebase Cloud Messaging (Google FCM) and PHP. In this, We are only going to discuss the PHP part.
What actually is a Push Notification?
A Push Notification is a message which is sent on the user’s smartphone. it is basically used to increase the engagement of users in-app. A push notification can be about anything whose main purpose is to let the user know about some events, offers, or anything, which reminds the user to open the app for that particular event or offer. By doing so the company increases the user engagement in their app by luring them with different offers sent by push notification(message).
Google Cloud Messaging
Google Cloud Messaging (GCM) is a free service provided by Google to enable developers to send messages from google server to app.
Google FCM
Later on, Google acquired Firebase in 2014 and then announced Firebase cloud messaging (FCM) to send notification and messages on different mobile OS even on iOS. FCM has lots of rich features which forces user to migrate from GCM to FCM.
Following are the steps you can configure PUSH notification from the website using Google FCM and PHP.
1 – Login/sign-up to your firebase account. https://console.firebase.google.com/
Select the project from the list or make a new one if you do not have any project there, It just asks some basic details about the app and can easily be created. If you are a backend developer then ask an android developer to create the same and provide you the necessary details.
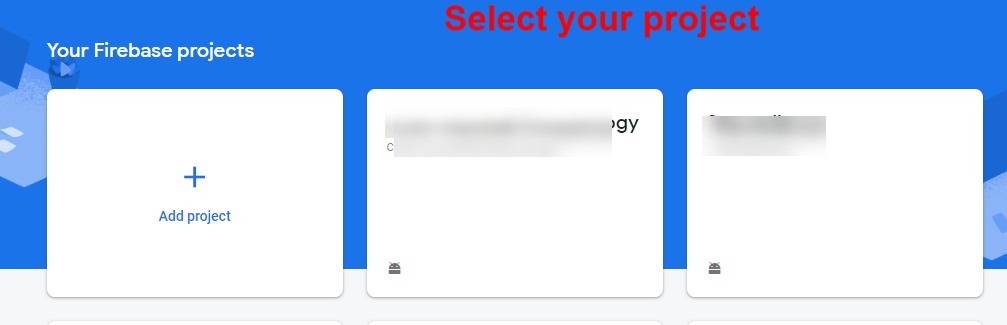
2 – Click on the gear icon on the left top side and then click ‘project settings.’
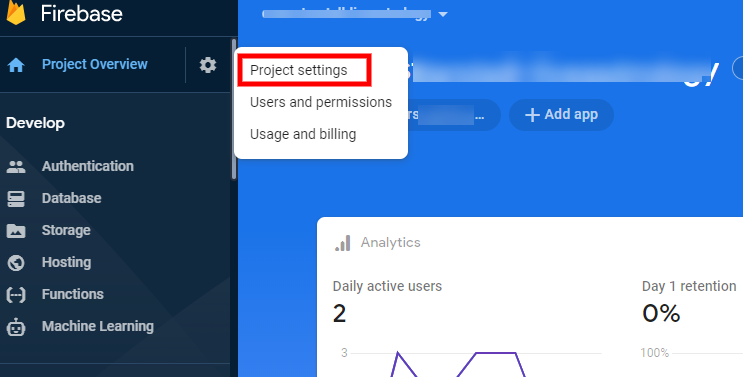
3 – Get the server key
Server key used for authentication when we send requests from our website to GCM server to send messages on mobile devices. Without the server key, you can not send the message.
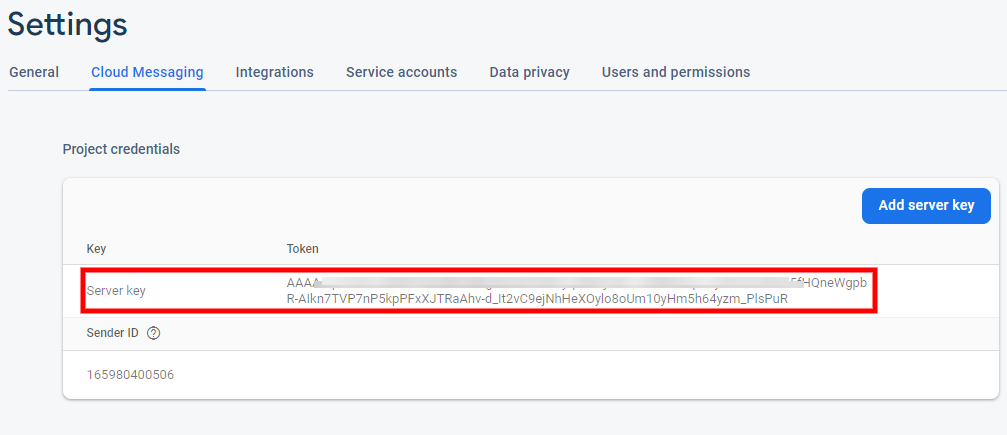
4 – Create notification.php
Create notification.php file and put the below code in it with valid credentials.
<?php //post data $notification_text = $_POST['notification_text']; $title = $_POST['title']; //you can insert post data into db for record // if you want to send data to single device $device_id ='fsdfsdfsdlj324j3l4j32l4jk2k4jldslkjflksdjfll23lk4j23'; // if you want to send data to multiple device $device_id = ['fsdfsdfsdlj324j3l4j32l4jk2k4jldslkjflksdjfll23lk4j23','fs423423dlj324j3l4j32l4jk2k4jldslkjflksdjfll23lk4j23']; // You can also fetch the data from database and put in array using foreach foreach($get_device_id as $all_device_id) { $device_id[] = $all_device_id->$device_id; // when data comes as object from DB } // FCM Payload data format $json_data = [ "registration_ids" => $device_id, "notification" => [ "body" => $notification_text, "title" => $title, ], "data" => [ "extra" => $customData // if you want for send extra data ] ]; $data = json_encode($json_data); // encode data into json format //FCM API end-point $url = 'https://fcm.googleapis.com/fcm/send'; //api_key in Firebase Console -> Project Settings -> CLOUD MESSAGING -> Server key $server_key = 'AAAAJqUz63o:APA91bH9dBz6RihgEdvKhi5dXJyqEl234234dfdf25--0ylOoQswedsseWgpbR-AIkn7TVP7nP5kpPFxXJTRaAhv-d_It2vC9ejNhHeXOylo8oUm10yHm5h64yzm_PlsPuR'; //header with content_type server key $headers = array( 'Content-Type:application/json', 'Authorization:key='.$server_key ); //CURL request to route notification to FCM connection server (provided by Google) $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_POSTFIELDS, $data); $result = curl_exec($ch); if ($result === FALSE) { die('Oops! FCM Send Error: ' . curl_error($ch)); } curl_close($ch); ?>
Hope it will help.
You can check How to send PUSH notification from the website using Google FCM and PHP in chunks. it is helpful when you need to send PUSH notification to more than 1k users, because FCM has limitation to send only 1k PUSH notification at a time.